-
Firstly, you need to know that Selenium supports five programming languages: Java, C#, Python, Ruby, Javascript (Node). Depending on language you use, there are different ways to set up proxies
-
Secondly, you need to choose WebDriver you want to use. Selenium offers WebDrivers to all the most popular browsers (you can see all supported drivers here). In our tutorial we'd like to use Google Chrome Driver
-
There are some code examples for your preferred programming language and Google Chrome Driver
package chromeScripts; import java.io.File; import org.openqa.selenium.By; import org.openqa.selenium.Proxy; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeDriverService; import org.openqa.selenium.chrome.ChromeOptions; public class Example { public static void main(String[] args) { String ProxyServer = "proxy.froxy.com"; int ProxyPort = 9000; String sHttpProxy = ProxyServer + ":" + ProxyPort; Proxy proxy = new Proxy(); proxy.setHttpProxy(sHttpProxy); ChromeDriverService service = new ChromeDriverService.Builder() .usingDriverExecutable(new File("PATH TO WEBDRIVER")) .usingAnyFreePort() .build(); ChromeOptions options = new ChromeOptions(); options.setCapability("proxy", proxy); options.merge(options); WebDriver driver=new ChromeDriver(service, options); driver.get("http://froxy.com/api/detect-ip/"); WebElement body = driver.findElement(By.tagName("body")); String bodyText = body.getText(); System.out.println(bodyText); } }
You're able to run all of these commands in your Terminal/Command Prompt window
-
To set up proxy connection please use proxy.froxy.com:9000 line within the punctuation marks (''). Depending on your type of language, punctuation may differ
- Java:
String ProxyServer = "proxy.froxy.com"; int ProxyPort = 9000;
- C#:
HttpProxy = "proxy.froxy.com:9000"
- Python:
HOSTNAME = 'proxy.froxy.com' PORT = '9000'
- Ruby:
proxy = Selenium::WebDriver::Proxy.new(http: "proxy.froxy.com:9000")
- Javascript (Node):
let addr = 'proxy.froxy.com:9000'
All necessary data about server, port, login and password you can get from your Froxy Dashboard
- Java:
Use proxies with Selenium
Learn how to set up resident and mobile proxies for test automation with Selenium.Choose Plan 3 day trial • No set-up costs • Cancel anytime
Pricing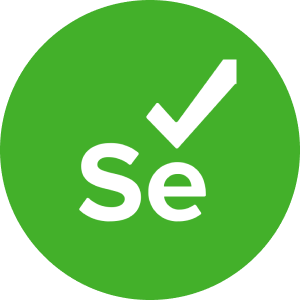
Try Froxy Right Now
Proxies from Froxy fit most popular tools that allow you to achieve your goals!
Try a proxy right now, just create an account, choose a rate and get started.
Start now